概要
カウントアップとカウントダウンを行うことができるアプリケーションです。
Vue.jsの導入にはCDNを用いています。
↓ ↓ ↓
最終挙動
サンプルコード
ディレクトリ構造
counter-app
- css
- style.css
- script
- script.js
- index.html
index.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="./css/style.css" />
<title>CounterApp</title>
</head>
<body>
<div id="counter">
<button id="increment-button" @click="increment">+ 1</button>
<button id="decrement-button" @click="decrement">- 1</button>
<p id="count" v-cloak>{{count}}</p>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue@2.6.12/dist/vue.js"></script>
<script src="./script/script.js"></script>
</body>
</html>
@clickはクリックイベントの省略系であり、原型は「v-on:click=”メソッド名”」となります。
{{…}}という記述はマスタッシュ構文と呼ばれ、Vueインスタンスのdata関数が返すオブジェクトのプロパティを紐付けることができます。
css/style.css
[v-cloak] {
visibility: hidden;
}
#counter {
display: flex;
align-items: center;
justify-content: space-around;
line-height: 600px;
}
#increment-button {
font-size: 30px;
font-weight: bold;
width: 300px;
height: 70px;
color: #000;
background-color: #eb6100;
border-bottom: 3px solid #d3d3d3;
border-radius: 5px;
}
#increment-button:hover {
margin-top: 3px;
color: #000;
background: #eb6100;
border-bottom: 2px solid #d3d3d3;
}
#decrement-button {
font-size: 30px;
font-weight: bold;
width: 300px;
height: 70px;
color: #000;
background-color: #f1e767;
border-bottom: 3px solid #ccc100;
border-radius: 5px;
}
#decrement-button:hover {
margin-top: 3px;
color: #000;
background: #f1e767;
border-bottom: 2px solid #ccc100;
}
#count {
font-size: 50px;
font-weight: bold;
text-align: center;
}
参考: CSSボタンデザイン120個以上!どこよりも詳しく作り方を解説!
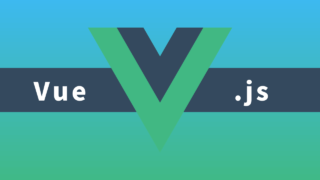
【Vue.js】コンパイル時に一瞬だけ表示されるマスタッシュ構文を隠す方法マスタッシュ構文で書かれたテンプレート変数を隠す方法について解説しています。コンパイルに時間がかかってしまい余計な変数が表示されてしまう現象はよく起こるため、参考にしていただけたらと思います。...
script/script.js
new Vue({
el: "#counter",
data() {
return {
count: 0
};
},
methods: {
increment() {
this.count++;
},
decrement() {
this.count--;
}
}
});
「this.count++;」という記述は「this.count = this.count – 1;」の省略系です。「this.count- -;」も同様です。