概要
カウントアップとカウントダウンを行うことができ、点数に応じてランクが変化するアプリケーションです。
- 3以上 → ランクA
- 0以上、3より小さい → ランクB
- 0より小さい → ランクC
Vue.jsの導入にはCDNを用いています。
↓ ↓ ↓
最終挙動
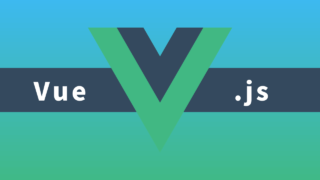
【Vue.js】カウンターアプリのサンプルコードVue.jsを用いてカウンターアプリを作成した際のサンプルコードを載せています。コピペOKですので、ご自身で色々カスタマイズしてみてください。...
サンプルコード
ディレクトリ構造
counter-app-2
- css
- style.css
- script
- script.js
- index.html
index.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="./css/style.css" />
<title>CounterApp2</title>
</head>
<body>
<div id="counter">
<button id="increment-button" @click="increment">+ 1</button>
<button id="decrement-button" @click="decrement">- 1</button>
<p id="count" v-cloak>{{count}}</p>
<p v-if="count >= 3" id="rank">ランクA</p>
<p v-else-if="count >=0" id="rank">ランクB</p>
<p v-else id="rank">ランクC</p>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue@2.6.12/dist/vue.js"></script>
<script src="./script/script.js"></script>
</body>
</html>
css/style.css
[v-cloak] {
visibility: hidden;
}
#counter {
display: flex;
align-items: center;
justify-content: space-around;
line-height: 600px;
}
#increment-button {
font-size: 30px;
font-weight: bold;
width: 300px;
height: 70px;
color: #000;
background-color: #eb6100;
border-bottom: 3px solid #d3d3d3;
border-radius: 5px;
}
#increment-button:hover {
margin-top: 3px;
color: #000;
background: #eb6100;
border-bottom: 2px solid #d3d3d3;
}
#decrement-button {
font-size: 30px;
font-weight: bold;
width: 300px;
height: 70px;
color: #000;
background-color: #f1e767;
border-bottom: 3px solid #ccc100;
border-radius: 5px;
}
#decrement-button:hover {
margin-top: 3px;
color: #000;
background: #f1e767;
border-bottom: 2px solid #ccc100;
}
#count,
#rank {
font-size: 50px;
font-weight: bold;
text-align: center;
}
script/script.js
new Vue({
el: "#counter",
data() {
return {
count: 0
};
},
methods: {
increment() {
this.count++;
},
decrement() {
this.count--;
}
}
});
算出プロパティを用いたコード
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="./css/style.css" />
<title>CounterApp2</title>
</head>
<body>
<div id="counter">
<button id="increment-button" @click="increment">+ 1</button>
<button id="decrement-button" @click="decrement">- 1</button>
<p id="count" v-cloak>{{count}}</p>
<p v-if="checkRank >= 3" id="rank">ランクA</p>
<p v-else-if="checkRank >= 0" id="rank">ランクB</p>
<p v-else id="rank">ランクC</p>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue@2.6.12/dist/vue.js"></script>
<script src="./script/script.js"></script>
</body>
</html>
new Vue({
el: "#counter",
data() {
return {
count: 0
};
},
methods: {
increment() {
this.count++;
},
decrement() {
this.count--;
}
},
computed: {
checkRank() {
return this.count;
}
}
});
[v-cloak] {
visibility: hidden;
}
#counter {
display: flex;
align-items: center;
justify-content: space-around;
line-height: 600px;
}
#increment-button {
font-size: 30px;
font-weight: bold;
width: 300px;
height: 70px;
color: #000;
background-color: #eb6100;
border-bottom: 3px solid #d3d3d3;
border-radius: 5px;
}
#increment-button:hover {
margin-top: 3px;
color: #000;
background: #eb6100;
border-bottom: 2px solid #d3d3d3;
}
#decrement-button {
font-size: 30px;
font-weight: bold;
width: 300px;
height: 70px;
color: #000;
background-color: #f1e767;
border-bottom: 3px solid #ccc100;
border-radius: 5px;
}
#decrement-button:hover {
margin-top: 3px;
color: #000;
background: #f1e767;
border-bottom: 2px solid #ccc100;
}
#count,
#rank {
font-size: 50px;
font-weight: bold;
text-align: center;
}